If you’re just starting out with JavaScript, you should know that it’s a great programming language for visualizing data. Data visualization is an extremely pertinent skill right now since we’re in the age of Big Data. Visualization tools are essential to make sense of it all and extract insights.
So here’s a basic rundown of how to create charts with JavaScript.
What You’ll Need to Create Charts with JavaScript
Internet Browser
The first thing you’ll need to implement any of the hundreds of Javascript solutions for charts is an internet browser. Chrome or Firefox are both fine for this exercise.
Text Editor
You’ll also need a text editor. Notepad will work fine, but you should ideally go for an advanced code editor like VS Code. It’ll give you a more convenient user experience which will make writing HTML5, CSS, Java Script, etc., easier. The code editor will help you find mistakes and correct them if your code doesn’t run.
Chart Library
Finally, you’ll need a chart library to generate the chart you need. I recommend using JSCharting, however, there are tons you can choose from. That being said, this tutorial will be for JSCharting. JSCharting can draw all sorts of charts with SVG and it’s easy to use. The API simplifies a lot of things and it’s a good option to experiment with when you’re beginning.
Data
Unless you have some data with you to generate a chart, you’ll need to get some. You can get data from places like the CDC, or WHO, or the WFP. Any data that pits two values against each other will do. Even charts that track the amount of rain in a city or a country per year is fine.
How to Create Charts with JavaScript
- Add a Blank Chart
You need to download a blank chart first with this. This file contains
- index.html
- js/index.js
The .html file is empty except for a little standard code. The .js file is completely empty.
- Open the index.html file in the code editor you’ve chosen and add a script tag to include the JSCharting library. Do this after the closing </body> tag.
The code should look like this:
</body>
<script src="https://code.jscharting.com/2.9.0/jscharting.js"></script>
</html>
- Then use the same technique to add a script tag to reference your blank .js file. Add it after the JSCharting script. It should look like this:
</body>
<script src="https://code.jscharting.com/2.9.0/jscharting.js"></script>
<script src="js/index.js"></script>
</html>
- Finally, add a <div> placeholder in the html file so that it defines where the chart is to be drawn.
It should look like this:
<body>
<div id="chartDiv" style="width:50%; height:300px; margin:0 auto;"></div>
</body>
- The div should have an ID so that it can tell the chart which div to draw in. Here, the ID is chartDiv.
Open the .js file and add a blank chart by writing the following code:
JSC.Chart('chartDiv', {});
- Finally, open the index.html file in a browser. You can drag and drop the file into a browser like chrome. You will be able to see a small JSC logo on the page. This is the first step complete to sending charts with Java script.
- Play Around a Little
Add a few values you can use to play around with the chart. Replace the content in the .js file with the following code:
JSC.Chart('chartDiv', {
type: 'horizontal column',
series: [
{
points: [
{x: 'Apples', y: 50},
{x: 'Oranges', y: 42}
]
}
]
});
- Refresh the browser where the page is loaded and you may see a bar chart. That’s because you set the type to ‘horizontal column’ in the code.
Now, let’s get down to business.
- Prepare Your Data Put it Together
- The CSV data format means comma separated values. This file contains rows, and each one represents a record or entry. The first row of values contains the names of the comma separated value. This is the column. Further rows contain values themselves. For example:
Name, age
Milton, 45
Harry, 34
- CSV is readable, but there are several variations of the format. If values contain commas, like mailing addresses, the format doesn’t work as is. Hence, each value has to be wrapped in quotes. Like this:
“name”, “age”
“Milton”, “45”
“Harry”, “34”
- JSCharting provides many tools to help with this. You can skip worrying about CSV and convert it to JSON (JavaScript Object Notation). This will be an array of objects.
First, download this file of CDC data for a sample project. You can open this CSV file in excel. You’ll need to download the CSV data in realtime with JavaScript code. The code can be a little confusing, but it’s short. Also, you can use it to get any CSV or JSON files over the internet. - Replace the index.js file content with this:
fetch('https://data.cdc.gov/resource/w9j2-ggv5.csv')
.then(function (response) {
return response.text();
})
.then(function (text) {
csvToSeries(text);
})
.catch(function (error) {
//Something went wrong
console.log(error);
});
function csvToSeries(text) {
console.log(text);
}
- The complex code makes sure that all the data is available immediately. That’s why it uses a fetch() function.
- The then(…) code is called for a response when it arrives. The function converts the response into text and then returns it.
- The second then(…) argument function passes the text as an argument.
- The catch() function specifies what to do if something goes wrong. This could be network problems, incorrect URLs, etc. In those cases, the error is sent to the console.
- After that the csvToSeries() function passes the text to the console for inspection.
- Putting it All Together
At the end of the index.js file, add this code to render the chart:
function renderChart(series){
JSC.Chart('chartDiv', {
series: series
});
}
Then refresh the browser, and you’ll have the chart that you need.
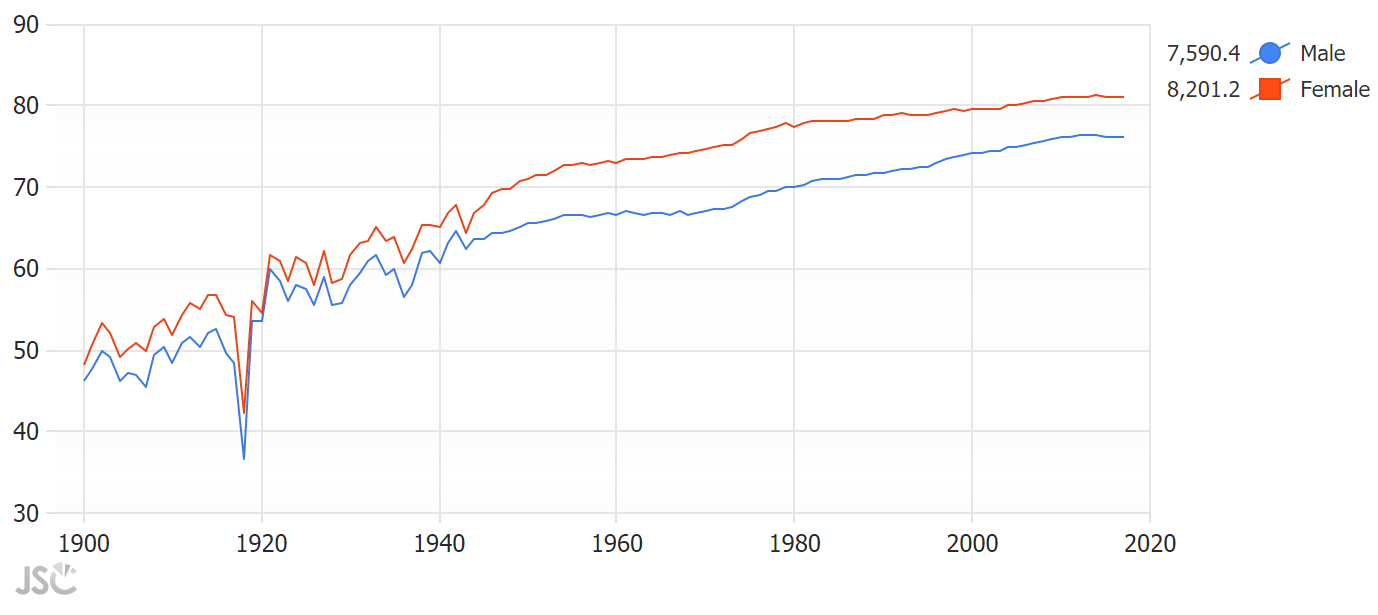
You can supplement your knowledge of java script charts with our article about bar charts.